728x90
참고자료: 유튜브 생활코딩 React 2022 개정판 https://www.youtube.com/playlist?list=PLuHgQVnccGMCOGstdDZvH41x0Vtvwyxu7
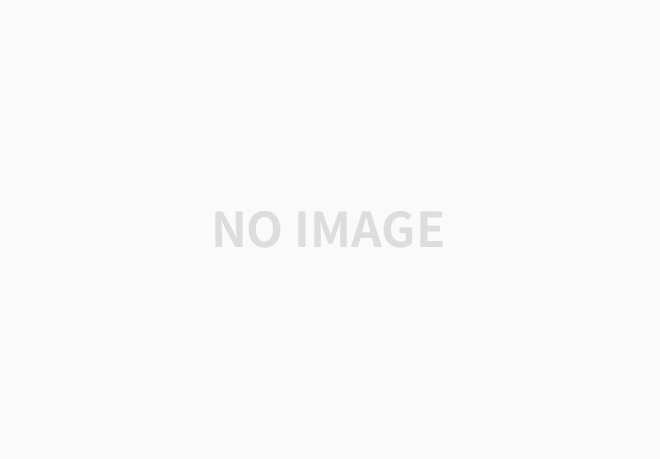
1. state
state값에 따라 article 내용 변경시키기
먼저 article 내용을 welcome과 read로 나눠 표시해보자!
import {useState} from 'react';
function App() {
const [mode, setMode] = useState("WELCOME");
const topics = [
{id : 1, title : 'html', body : 'html is ...'},
{id : 2, title : 'css', body : 'css is ...'},
{id : 3, title : 'js', body : 'js is ...'},
]
let content = null;
if (mode === "WELCOME") {
content = <Article title="Welcome" body="Hello, WEB!"></Article>
} else if (mode === "READ") {
content = <Article title="Read" body="Hello, Read!"></Article>
}
return (
<div>
<Header title="WEB" onChangeMode={()=>{
setMode("WELCOME");
}}></Header>
<Nav topics={topics} onChangeMode={(id)=>{
setMode("READ");
}}></Nav>
{content}
</div>
);
}
👉🏻전체 코드는 이렇다.
import {useState} from 'react';
const [mode, setMode] = useState("WELCOME");
👉🏻먼저 state를 활용하기 위해 useState를 import한다.
👉🏻useState를 활용하면 state의 초깃값인 배열을 리턴한다. 배열 0번째에는 state 값이 들어있고 1번째에는 state인 mode의 값을 바꿀 수 있다.
return (
<div>
<Header title="WEB" onChangeMode={()=>{
setMode("WELCOME");
}}></Header>
<Nav topics={topics} onChangeMode={(id)=>{
setMode("READ");
}}></Nav>
{content}
</div>
);
👉🏻return 부분에서 Nav 컴포넌트나 Header 컴포넌트를 누르면 setMode가 호출되면서 App이 다시 실행되어 mode가 인자값으로 바뀐다!
let content = null;
if (mode === "WELCOME") {
content = <Article title="Welcome" body="Hello, WEB!"></Article>
} else if (mode === "READ") {
content = <Article title="Read" body="Hello, Read!"></Article>
}
👉🏻그리고 변경된 mode의 값에 따라 content에 다른 props를 가진 Article 컴포넌트를 넣어준다.
return (
<div>
...중략...
{content}
</div>
);
👉🏻그리고 content를 return해주면 된다!
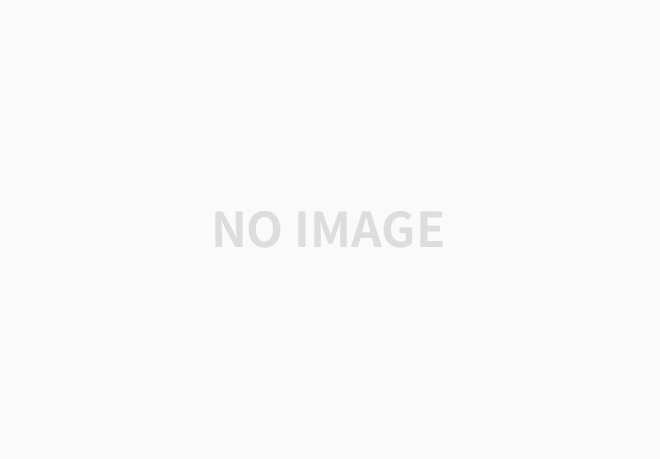
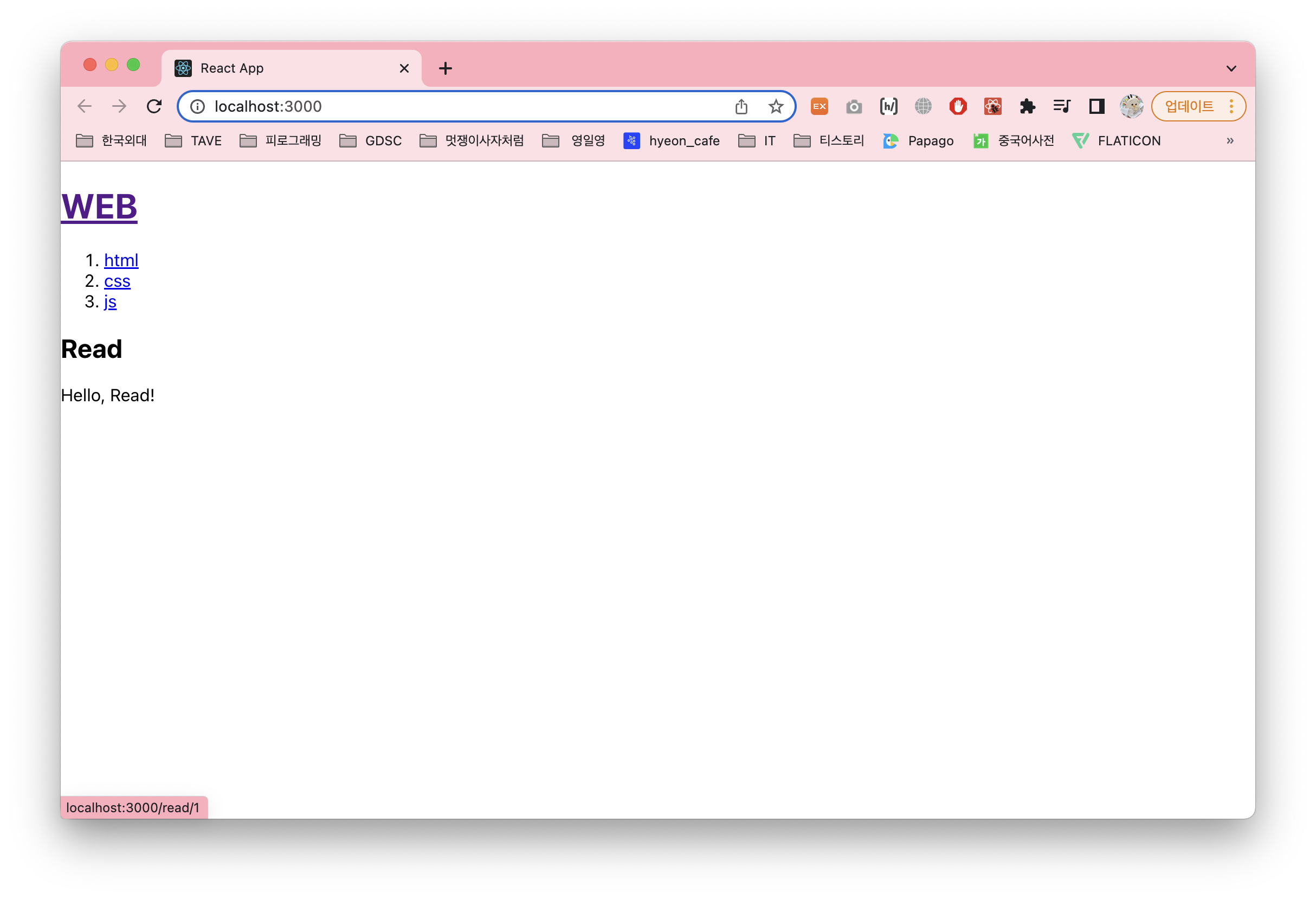
이제 article 내용을 Nav 컴포넌트에서 클릭한 내용에 맞게 변하게 하자!
function App() {
const [mode, setMode] = useState("WELCOME");
const [id, setId] = useState(null);
const topics = [
{id : 1, title : 'html', body : 'html is ...'},
{id : 2, title : 'css', body : 'css is ...'},
{id : 3, title : 'js', body : 'js is ...'},
]
let content = null;
if (mode === "WELCOME") {
content = <Article title="Welcome" body="Hello, WEB!"></Article>
} else if (mode === "READ") {
let title, body = null;
for(let i = 0; i < topics.length; i++){
if(topics[i].id === id) {
title = topics[i].title;
body = topics[i].body;
}
}
content = <Article title={title} body={body}></Article>
}
return (
<div>
<Header title="WEB" onChangeMode={()=>{
setMode("WELCOME");
}}></Header>
<Nav topics={topics} onChangeMode={(_id)=>{
setMode("READ");
setId(_id);
}}></Nav>
{content}
</div>
);
}
👉🏻이것이 전체코드!
const [id, setId] = useState(null);
👉🏻먼저 id라는 state를 null로 설정한다.
return (
<div>
...중략...
<Nav topics={topics} onChangeMode={(_id)=>{
setMode("READ");
setId(_id);
}}></Nav>
{content}
</div>
);
👉🏻Nav 컴포넌트를 return하는 부분에서 setId를 이용해 클릭된 요소의 Id값을 넣어준다.
let content = null;
if (mode === "WELCOME") {
content = <Article title="Welcome" body="Hello, WEB!"></Article>
} else if (mode === "READ") {
let title, body = null;
for(let i = 0; i < topics.length; i++){
if(topics[i].id === id) {
title = topics[i].title;
body = topics[i].body;
}
}
content = <Article title={title} body={body}></Article>
}
👉🏻그리고 for문을 돌리면서 받아온 id 값과 같은 id값을 가진 topics 객체를 골라 Article 컴포넌트 props를 채워준다.


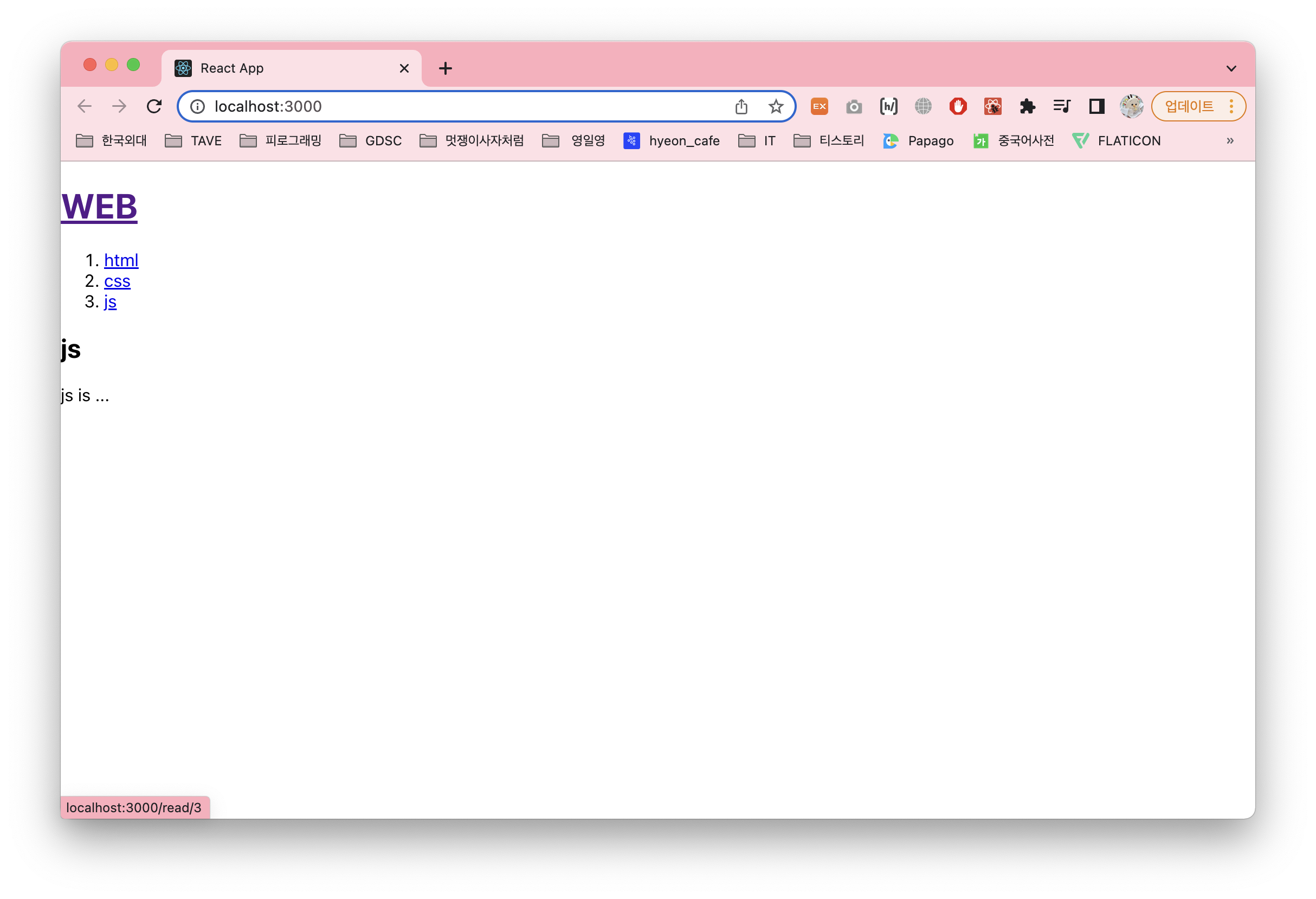
728x90
'그 땐 Front했지 > 그 땐 React했지' 카테고리의 다른 글
[생활코딩/React] 2022개정판 | 9. update (0) | 2022.05.06 |
---|---|
[생활코딩/React] 2022개정판 | 8. create (0) | 2022.05.05 |
[생활코딩/React] 2022개정판 | 6. 이벤트 (0) | 2022.05.02 |
[생활코딩/React] 2022개정판 | 4. 컴포넌트 만들기 (0) | 2022.05.02 |
[생활코딩/React] 2022개정판 | 5. props (0) | 2022.05.02 |